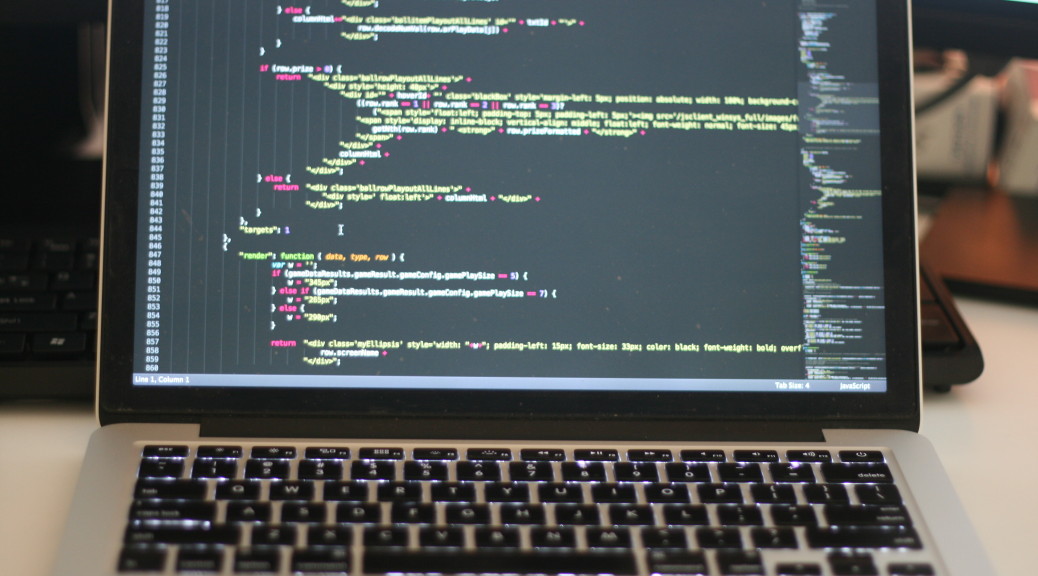
How to jump from ActionScript to Javascript / HTML5
When I started thinking about this subject I thought maybe it was just me, in my particular situation and work experience, who got left behind web technologies. But after conversations with many senior developers, I concluded this is a pretty common theme among us and a problem worth tackling.
The purpose of this article is to shed some light onto web technologies from a principles point of view. This is targeted to senior developers who have experience in more formal languages such as as3, C++, Java, Assembly(!!). It is also targeted for CTOs, Business Managers, Project Managers and any other decision making personnel who is having a tough time with the terminology. Since I’ve been coding mostly in ActionScript for the past years, I will use it as a reference in this article, but the general concepts apply to other programming languages as well. And although there are many technology stacks used today to create web applications, I will focus on JavaScript related ones because those are the ones I am currently learning. Of course, the concepts can be extrapolated to other technologies (such as PHP, .NET, Ruby, etc). Please be aware, this is not a technical article. You will not learn how to migrate your code from as3 to JavaScript. This is one level of abstraction higher
When someone is looking to start working on a web-app, they will quickly be bombarded with the following terminology: JavaScript, HTML, css, css3, DOM, jQuery, Ember, Angular, Angular2, React, Node, MEAN Stack, Full-Stack, MongoDB, Bootstrap, Responsive, Mobile-Ready, ES6, ES7, TypeScript, Redux, DevOps, JSON, XML, Webpack, Stringify. Enough terminology to fill out a resume! Someone who wrote physics engines for AAA games might get completely overwhelmed by this.
But don’t be afraid, you are not alone, and it’s perfectly normal to be able to write a full blow car physics algorithm and not to have a clue how to write a “hello world” web page. For those who started the proper way (from basic HTML to older AJAX techniques and then dived into exotic new frameworks such as AngularJS), I ask you this: Imagine learning Angular without knowing what a <div> is. How would you feel?
The reverse is also true: imagine doing a physics engine in C++ without understanding pointers and recursion.
Before we move forward into web application structure, let’s point out one of the biggest differences: there’s no compile!
We are used to the following flow:
- Write Code
- Compile
- Run
- Debug
With web apps, you simply write code, then you refresh the webpage. The latter step being sometimes a combination of running and debugging. And depending on which technologies you use, the refreshing is also optional. So you end up writing code and seeing the changes live. How cool is that?
So here is a list of important key concepts which helped me jump ship from as3 to web apps:
1. You are building HTML. Always!
This is one thing which, although it seems extremely trivial now, it wasn’t easy to grasp in the beginning. When using ANY web technologies your end result is HTML. Everything you do involves creating, manipulating and styling HTML components, also called DOM nodes.
You might be somewhat familiar with <head>, <body> of an html site, but the building blocks of the layout are really divs, tables, lists, buttons and input fields.
There is a ton of documentation on the ins and outs of this, but the basic principle is this: your end result is a web page, made up of HTML tags. You simply use Javascript and other frameworks to manipulate those tags. That’s all.
2. JavaScript is your best friend
So you are building HTML and you need something to manipulate it with – enter JavaScript. If you come from a more traditional language such as C++, you will probably feel like puking at this thought. But have no fear, it’s just how things work. (Why C++ developers should not be scared of JavaScript)
JavaScript is a lot more powerful than people give it credit. JavaScript can be Procedural, Structured, Object-Oriented, Functional, Event-Driven and Interpreted. It all depends on how you use it. Also, passing functions as arguments is an awesome way to do some really crazy things! Also, JavaScript is fast!
3. Goolge Chrome is your other best friend
One of the most difficult things to wrap your head around web application development is the dev environment. You might be used to Eclipse, Flash Builder, Visual Studio – and all of the sudden all the examples you see are done in Notepad++ at best, no compile, and just browser refresh.
This will take some getting used to, so I highly recommend trying out different setups. The two most common editors are Sublime Text and Webstorm. Bonus points if you write a full stack app using vi only 🙂
I also recommend getting very familiar with Google Chrome’s developer tools, as they will provide tremendous help when developing and debugging.
4. Social Network is the new “Hello World” app.
It is widely acceptable to jump into a new programming language or technology by writing a “hello world” program. This is not good enough anymore. The new “hello world” should be a fully functional social network (including deployment to a cloud based service Amazon Web Services or MS Azure). I did mine in 30 hours, so if you skip on endless Facebook scrolling, you can do too during a long weekend!
The social network should have at a minimum the following:
Stage 1:
- Landing page for sign-in or sign up
- Create/Edit profile
- Make friends
Stage 2:
- live, real time encrypted chat module
Stage 3:
- Native iPhone, Android and Windows Mobile apps.
Stage 4:
- backoffice / admin tool for the social network.
- This tool should have the capability to see stats of the system, ban users, send mass messages, remove accounts, etc.
Although you are not expected to be a designer, the app should not look like it was put together by a developer. There are many styles of UI components which look very nice and could be used. If you are a developer, it doesn’t mean you can’t create good looking apps without the input of a designer.
Creating and deploying a Social Network app will make you understand all aspects of the technologies used. And although you will not become a database guru, you will at least have an idea of what it means to persist data to a database and how to retrieve it and what technologies to use.
I always believed that great products are built by great teams, not by great skills. Anybody has the ability to improve their technical skills and there will ALWAYS be a better solution and room for improvement. What differentiates good products from mediocre ones is how well the team works together. A team of average coders that get along very well will put together a much better product than a team of elite coders that don’t get along.
5. Choosing the right technologies – chose all!
One of the most daunting tasks for a CTO or software developer when starting a new project is to chose which web technology to use. There are so many! And so many combinations! The best way to decide is to create a series of small pet projects and use different technologies for different ones.
For example, we can take our social network example and first build the backend in Node.js and then build the same functionality in Ruby. This would be an excellent exercise to compare multiple technologies. You can then dump the code in github and show to potential employers that you wrote open source software 🙂
6. Understanding the lingo
Above, I listed most of the key words which are currently hot in the web development world. I will explain to them in simple, plain english, so that you understand how they all fit together:
JavaScript, HTML, css, css3, DOM, jQuery, Ember, Angular, Angular2, React, Node, MongoDB, MEAN Stack, Full-Stack, MongoDB, Bootstrap, Responsive, Mobile-Ready, ES6, ES7, TypeScript, Redux, DevOps, JSON, XML, Webpack, Stringif
You are building web applications. The end result is HTML. You use the JavaScript to manipulate the DOM (HTML) and to write your business logic. The application can be broken down into 2 distinct areas: the UI and the logic.
The UI is how the app looks – colors, styles, fonts and animations. These are controled by css (css3) bootstrap and jQuery.
The logic is written in JavaScript, but it can’t all be dumped in one file. So we use frameworks. Frameworks include: Angular/Angular2, React/Redux. Ember.
7. Bring value to the customer!
The most important lesson is by far bringing value to the customer. This goes beyond programming and software engineering. This is the thing that should pop in everyone’s head when thinking about a new product. Why are we migrating from Flex to html5? or Why are so many companies re-writing their software? Because the end-user requirements change constantly.
When making the decision of which framework to use, besides the technical details or requirements, a CTO should also keep in mind the available work force for hire. At the time I’m writing this article, React / Redux is a very cool and funky new framework for developing web apps created and backed by Facebook, but it would be a pretty dumb idea to create a product using it if you expect to hire 10 more programmers next year. The reason is you won’t have anybody to choose from!
Another value you can bring to the customer using web technologies is the long term maintainability of the code. Since all these technologies work interchangeably with one another, it is very important to ask the following questions:
- How easy is it to re-write part of the app in the future? (the UI, the back-end, the database)
- Can I easily find programmers to maintain my code in 2 years from now?
Conclusions
I hope I shined some light on some of the questions regarding HTML5 migration and web applications in general. As you can see, switching from classic application development to web applications goes way beyond learning JavaScript and HTML. Those are just simple, implementation details which can be picked up in a few weeks by a seasoned developer. The real value is understanding well the above points, and I hope this article will open many doors to many developers which are in doubt about jumping ship to web application development.




